- 积分
- 24
- 威望
-
- 金钱
-
- 注册时间
- 2012-6-7
- 在线时间
- 小时
- 最后登录
- 1970-1-1
|
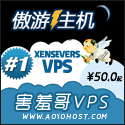 - #!/usr/bin/env bash
- #
- # Dropbox Uploader
- #
- # Copyright (C) 2010-2013 Andrea Fabrizi
- #
- # This program is free software; you can redistribute it and/or modify
- # it under the terms of the GNU General Public License as published by
- # the Free Software Foundation; either version 2 of the License, or
- # (at your option) any later version.
- #
- # This program is distributed in the hope that it will be useful,
- # but WITHOUT ANY WARRANTY; without even the implied warranty of
- # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
- # GNU General Public License for more details.
- #
- # You should have received a copy of the GNU General Public License
- # along with this program; if not, write to the Free Software
- # Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
- #
- #Default configuration file
- CONFIG_FILE=~/.dropbox_uploader
- #If you are experiencing problems establishing SSL connection with the DropBox
- #server, try to uncomment this option.
- #Note: This option explicitly allows curl to perform "insecure" SSL connections and transfers.
- #CURL_ACCEPT_CERTIFICATES="-k"
- #Default chunk size in Mb for the upload process
- #It is recommended to increase this value only if you have enough free space on your /tmp partition
- #Lower values may increase the number of http requests
- CHUNK_SIZE=4
- #Set to 1 to enable DEBUG mode
- DEBUG=0
- #Set to 1 to enable VERBOSE mode
- VERBOSE=1
- #Curl location
- #If not set, curl will be searched into the $PATH
- #CURL_BIN="/usr/bin/curl"
- #Temporary folder
- TMP_DIR="/tmp"
- #Don't edit these...
- API_REQUEST_TOKEN_URL="https://api.dropbox.com/1/oauth/request_token"
- API_USER_AUTH_URL="https://www2.dropbox.com/1/oauth/authorize"
- API_ACCESS_TOKEN_URL="https://api.dropbox.com/1/oauth/access_token"
- API_CHUNKED_UPLOAD_URL="https://api-content.dropbox.com/1/chunked_upload"
- API_CHUNKED_UPLOAD_COMMIT_URL="https://api-content.dropbox.com/1/commit_chunked_upload"
- API_UPLOAD_URL="https://api-content.dropbox.com/1/files_put"
- API_DOWNLOAD_URL="https://api-content.dropbox.com/1/files"
- API_DELETE_URL="https://api.dropbox.com/1/fileops/delete"
- API_MOVE_URL="https://api.dropbox.com/1/fileops/move"
- API_METADATA_URL="https://api.dropbox.com/1/metadata"
- API_INFO_URL="https://api.dropbox.com/1/account/info"
- API_MKDIR_URL="https://api.dropbox.com/1/fileops/create_folder"
- API_SHARES_URL="https://api.dropbox.com/1/shares"
- APP_CREATE_URL="https://www2.dropbox.com/developers/apps"
- RESPONSE_FILE="$TMP_DIR/du_resp_$RANDOM"
- CHUNK_FILE="$TMP_DIR/du_chunk_$RANDOM"
- BIN_DEPS="sed basename date grep stat dd printf mkdir"
- VERSION="0.11.8"
- umask 077
- #Check the shell
- if [ -z "$BASH_VERSION" ]; then
- echo -e "Error: this script requires BASH shell!"
- exit 1
- fi
- if [ $DEBUG -ne 0 ]; then
- set -x
- RESPONSE_FILE="$TMP_DIR/du_resp_debug"
- fi
- #Look for optional config file parameter
- while getopts ":f:" opt; do
- case $opt in
- f)
- CONFIG_FILE=$OPTARG
- shift $((OPTIND-1))
- ;;
- \?)
- echo "Invalid option: -$OPTARG" >&2
- exit 1
- ;;
-
- echo "Option -$OPTARG requires an argument." >&2
- exit 1
- ;;
- esac
- done
- #Print verbose information depends on $VERBOSE variable
- function print
- {
- if [ $VERBOSE -eq 1 ]; then
- echo -ne "$1";
- fi
- }
- #Returns unix timestamp
- function utime
- {
- echo $(date +%s)
- }
- #Remove temporary files
- function remove_temp_files
- {
- if [ $DEBUG -eq 0 ]; then
- rm -fr "$RESPONSE_FILE"
- rm -fr "$CHUNK_FILE"
- fi
- }
- #Returns the file size in bytes
- # generic GNU Linux: linux-gnu
- # windows cygwin: cygwin
- # raspberry pi: linux-gnueabihf
- # macosx: darwin10.0
- # freebsd: FreeBSD
- # qnap: linux-gnueabi
- # iOS: darwin9
- function file_size
- {
- #Some embedded linux devices
- if [ "$OSTYPE" == "linux-gnueabi" -o "$OSTYPE" == "linux-gnu" ]; then
- stat -c "%s" "$1"
- return
- #Generic Unix
- elif [ "${OSTYPE:0:5}" == "linux" -o "$OSTYPE" == "cygwin" -o "${OSTYPE:0:7}" == "solaris" -o "${OSTYPE}" == "darwin9" ]; then
- stat --format="%s" "$1"
- return
- #BSD or others OS
- else
- stat -f "%z" "$1"
- return
- fi
- }
- #USAGE
- function usage
- {
- echo -e "Dropbox Uploader v$VERSION"
- echo -e "Andrea Fabrizi - andrea.fabrizi@gmail.com\n"
- echo -e "Usage: $0 COMMAND [PARAMETERS]..."
- echo -e "\nCommands:"
- echo -e "\t upload [LOCAL_FILE/DIR] "
- echo -e "\t download [REMOTE_FILE/DIR] "
- echo -e "\t delete [REMOTE_FILE/DIR]"
- echo -e "\t move [REMOTE_FILE/DIR] [REMOTE_FILE/DIR]"
- echo -e "\t mkdir [REMOTE_DIR]"
- echo -e "\t list "
- echo -e "\t share [REMOTE_FILE]"
- echo -e "\t info"
- echo -e "\t unlink"
- echo -en "\nFor more info and examples, please see the README file.\n\n"
- remove_temp_files
- exit 1
- }
- if [ -z "$CURL_BIN" ]; then
- BIN_DEPS="$BIN_DEPS curl"
- CURL_BIN="curl"
- fi
- #DEPENDENCIES CHECK
- for i in $BIN_DEPS; do
- which $i > /dev/null
- if [ $? -ne 0 ]; then
- echo -e "Error: Required program could not be found: $i"
- remove_temp_files
- exit 1
- fi
- done
- #Urlencode
- function urlencode
- {
- local string="${1}"
- local strlen=${#string}
- local encoded=""
- for (( pos=0 ; pos<strlen ;="" pos++="" ));="" do
- c=${stringpos:1}
- case "$c" in
- [-_.~a-zA-Z0-9] ) o="${c}" ;;
- * ) printf -v o '%%%02x' "'$c"
- esac
- encoded+="${o}"
- done
- echo "$encoded"
- }
- #Generic upload wrapper around db_upload_file and db_upload_dir functions
- #$1 = Local source file/dir
- #$2 = Remote destination file/dir
- function db_upload
- {
- local SRC="$1"
- local DST="$2"
- #It's a file
- if [ -f "$SRC" ]; then
- db_upload_file "$SRC" "$DST"
-
- #It's a directory
- elif [ -d "$SRC" ]; then
- db_upload_dir "$SRC" "$DST"
- #Unsupported object...
- else
- print " > Skipping not regular file '$SRC'"
- fi
- }
- #Generic upload wrapper around db_chunked_upload_file and db_simple_upload_file
- #The final upload function will be choosen based on the file size
- #$1 = Local source file
- #$2 = Remote destination file
- function db_upload_file
- {
- local FILE_SRC="$1"
- local FILE_DST="$2"
- #Checking file size
- FILE_SIZE=$(file_size "$FILE_SRC")
- #Checking the free quota
- FREE_QUOTA=$(db_free_quota)
- if [ $FILE_SIZE -gt $FREE_QUOTA ]; then
- let FREE_MB_QUOTA=$FREE_QUOTA/1024/1024
- echo -e "Error: You have no enough space on your DropBox!"
- echo -e "Free quota: $FREE_MB_QUOTA Mb"
- remove_temp_files
- exit 1
- fi
- if [ $FILE_SIZE -gt 157286000 ]; then
- #If the file is greater than 150Mb, the chunked_upload API will be used
- db_chunked_upload_file "$FILE_SRC" "$FILE_DST"
- else
- db_simple_upload_file "$FILE_SRC" "$FILE_DST"
- fi
- }
- #Simple file upload
- #$1 = Local source file
- #$2 = Remote destination file
- function db_simple_upload_file
- {
- local FILE_SRC="$1"
- local FILE_DST=$(urlencode "$2")
- ........................
-
复制代码 代码权限:chmod +x dropbox_uploader.sh
网上说的用这个 sudo dpkg-reconfigure dash 修改代码执行方式,但是执行过后就提示
错误代码:- root@bcsytv:~# sh dropbox_uploader.sh
- dropbox_uploader.sh: dropbox_uploader.sh: cannot execute binary file
复制代码 程序版本是ubuntu 13.04
[ 本帖最后由 jalena 于 2013-6-20 20:09 编辑 ] |
|